图书介绍
INTRODUCTION TO PROGRAMMING WITH C++【2025|PDF下载-Epub版本|mobi电子书|kindle百度云盘下载】
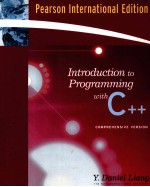
- Y.DANIEL LIANG 著
- 出版社: PEARSON PRENTICE HALL
- ISBN:
- 出版时间:2007
- 标注页数:661页
- 文件大小:156MB
- 文件页数:663页
- 主题词:
PDF下载
下载说明
INTRODUCTION TO PROGRAMMING WITH C++PDF格式电子书版下载
下载的文件为RAR压缩包。需要使用解压软件进行解压得到PDF格式图书。建议使用BT下载工具Free Download Manager进行下载,简称FDM(免费,没有广告,支持多平台)。本站资源全部打包为BT种子。所以需要使用专业的BT下载软件进行下载。如BitComet qBittorrent uTorrent等BT下载工具。迅雷目前由于本站不是热门资源。不推荐使用!后期资源热门了。安装了迅雷也可以迅雷进行下载!
(文件页数 要大于 标注页数,上中下等多册电子书除外)
注意:本站所有压缩包均有解压码: 点击下载压缩包解压工具
图书目录
PART 1 FUNDAMENTALS OF PROGRAMMING19
Chapter 1 Introduction to Computers,Programs, and C++21
1.1 Introduction22
1.2 What Is a Computer?22
1.3 Programs25
1.4 Operating Systems27
1.5 (Optional) Number Systems28
1.6 History of C++31
1.7 A Simple C++ Program32
1.8 C++ Program Development Cycle33
1.9 Developing C++ Programs Using Visual C++35
1.10 Developing C++ Programs Using Dev-C++41
1.11 Developing C++ Programs from Command Line on Windows46
1.12 Developing C++ Programs on UNIX47
Chapter 2 Primitive Data Types and Operations51
2.1 Introduction52
2.2 Writing Simple Programs52
2.3 Reading Input from the Keyboard54
2.4 Omitting the std: Prefix55
2.5 Identifiers56
2.6 Variables56
2.7 Assignment Statements and Assignment Expressions57
2.8 Named Constants59
2.9 Numeric Data Types and Operations60
2.10 Numeric Type Conversions66
2.11 Character Data Type and Operations68
2.12 Case Studies70
2.13 Programming Style and Documentation75
2.14 Programming Errors76
2.15 Debugging77
Chapter 3 Selection Statements85
3.1 Introduction86
3.2 The bool Data Type86
3.3 i f Statements87
3.4 Example: Guessing Birth Dates89
3.5 Logical Operators91
3.6 if.else Statements94
3.7 Nested i f Statements95
3.8 Example: Computing Taxes97
3.9 Example: A Math Learning Tool100
3.10 swi tch Statements101
3.11 Conditional Expressions104
3.12 Formatting Output104
3.13 Operator Precedence and Associativity107
3.14 Enumerated Types109
Chapter 4 Loops119
4.1 Introduction120
4.2 The while Loop120
4.3 The do-while Loop124
4.4 The for Loop126
4.5 Which Loop to Use?129
4.6 Nested Loops130
4.7 Case Studies131
4.8 (Optional) Keywords break and continue136
4.9 Example: Displaying Prime Numbers138
4.10 (Optional) Simple File Input and Output140
Chapter 5 Functions153
5.1 Introduction154
5.2 Creating a Function154
5.3 Calling a Function155
5.4 void Functions157
5.5 Passing Parameters by Values159
5.6 Passing Parameters by References161
5.7 Overloading Functions163
5.8 Function Prototypes165
5.9 Default Arguments167
5.10 Case Study: Computing Taxes with Functions168
5.11 Reusing Functions by Different Programs170
5.12 Case Study: Generating Random Characters171
5.13 The Scope of Variables173
5.14 The Math Functions177
5.15 Function Abstraction and Stepwise Refinement177
5.16 (Optional) Inline Functions185
Chapter 6 Arrays199
6.1 Introduction200
6.2 Array Basics200
6.3 Passing Arrays to Functions207
6.4 Returning Arrays from Functions210
6.5 Searching Arrays212
6.6 Sorting Arrays215
6.7 Two-Dimensional Arrays218
6.8 (Optional) Multidimensional Arrays225
Chapter 7 Pointers and C-Strings237
7.1 Introduction238
7.2 Pointer Basics238
7.3 Passing Arguments by References with Pointers241
7.4 Arrays and Pointers242
7.5 Using const with Pointers244
7.6 Returning Pointers from Functions245
7.7 Dynamic Memory Allocation247
7.8 Case Studies: Counting the Occurrences of Each Letter249
7.9 Characters and Strings252
7.10 Case Studies: Checking Palindromes260
Chapter 8 Recursion269
8.1 Introduction270
8.2 Example: Factorials270
8.3 Example: Fibonacci Numbers272
8.4 Problem Solving Using Recursion274
8.5 Recursive Helper Functions276
8.6 Towers of Hanoi279
8.7 Recursion versus Iteration282
PART 2 OBJECT-ORIENTED PROGRAMMING289
Chapter 9 Objects and Classes291
9.1 Introduction292
9.2 Defining Classes for Objects292
9.3 Constructors294
9.4 Object Names294
9.5 Separating Declaration from Implementation298
9.6 Accessing Object Members via Pointers300
9.7 Creating Dynamic Objects on Heap301
9.8 The C++ string Class301
9.9 Data Field Encapsulation305
9.10 The Scope of Variables308
9.11 The this Pointer310
9.12 Passing Objects to Functions311
9.13 Array of Objects313
9.14 Class Abstraction and Encapsulation315
9.15 Case Study: The Loan Class315
9.16 Constructor Initializer Lists319
Chapter 10 More on Objects and Classes329
10.1 Introduction330
10.2 Immutable Objects and Classes330
10.3 Preventing Multiple Declarations332
10.4 Instance and Static Members334
10.5 Destructors337
10.6 Copy Constructors339
10.7 Customizing Copy Constructors342
10.8 fri end Functions and fri end Classes344
10.9 Object Composition346
10.10 Case Study: The Course Class347
10.11 Case Study: The StackOfIntegers Class350
10.12 The C++ vector Class353
Chapter 11 Inheritance and Polymorphism361
11.1 Introduction362
11.2 Base Classes and Derived Classes362
11.3 Generic Programming368
11.4 Constructors and Destructors368
11.5 Redefining Functions371
11.6 Polymorphism and Virtual Functions372
11.7 The protected Keyword375
11.8 Abstract Classes and Pure Virtual Functions376
11.9 Dynamic Casting380
Chapter 12 File Input and Output391
12.1 Introduction392
12.2 Text I/O392
12.3 Formatting Output396
12.4 Member Functions: getline, get, and put397
12.5 f stream and File Open Modes400
12.6 Testing Stream States401
12.7 Binary I/O403
12.8 Random Access File410
12.9 Updating Files413
Chapter 13 Operator Overloading417
13.1 Introduction418
13.2 The Rational Class418
13.3 Operator Functions423
13.4 Overloading the Shorthand Operators425
13.5 Overloading the [] Operators425
13.6 Overloading the Unary Operators427
13.7 Overloading the ++ and —— Operators427
13.8 Overloading the << and >> Operators428
13.9 Object Conversion430
13.10 The New Rational Class431
13.11 Overloading the = Operators438
Chapter 14 Exception Handling443
14.1 Introduction444
14.2 Exception-Handling Overview444
14.3 Exception-Handling Advantages446
14.4 Exception Classes447
14.5 Custom Exception Classes450
14.6 Multiple Catches455
14.7 Exception Propagation458
14.8 Rethrowing Exceptions460
14.9 Exception Specification461
14.10 When to Use Exceptions462
PART 3 DATA STRUCTURES467
Chapter 15 Templates469
15.1 Introduction470
15.2 Templates Basics470
15.3 Example: A Generic Sort472
15.4 Class Templates474
15.5 Improving the Stack Class480
Chapter 16 Linked Lists, Stacks, and Queues487
16.1 Introduction488
16.2 Nodes488
16.3 The Li nkedLi st Class489
16.4 Implementing Li nkedLi st492
16.5 Variations of Linked Lists502
16.6 Implementing Stack Using a Li nkedLi st502
16.7 Queues504
16.8 (Optional) Iterators506
Chapter 17 Trees, Heaps, and Priority Queues515
17.1 Introduction516
17.2 Binary Trees516
17.3 Heaps524
17.4 Priority Queues529
Chapter 18 Algorithm Efficiency and Sorting535
18.1 Introduction536
18.2 Estimating Algorithm Efficiency536
18.3 Bubble Sort541
18.4 Merge Sort543
18.5 Quick Sort547
18.6 Heap Sort551
18.7 External Sort552
热门推荐
- 1030911.html
- 1825112.html
- 3032721.html
- 3484419.html
- 2360429.html
- 2366649.html
- 856551.html
- 2357920.html
- 3824343.html
- 942186.html
- http://www.ickdjs.cc/book_2336915.html
- http://www.ickdjs.cc/book_1146242.html
- http://www.ickdjs.cc/book_203852.html
- http://www.ickdjs.cc/book_188764.html
- http://www.ickdjs.cc/book_1836205.html
- http://www.ickdjs.cc/book_2706534.html
- http://www.ickdjs.cc/book_201772.html
- http://www.ickdjs.cc/book_1327729.html
- http://www.ickdjs.cc/book_1999897.html
- http://www.ickdjs.cc/book_2711542.html